Code Like a Pro: Best Practices for Writing Clean and Efficient Code
In the ever-evolving world of programming, writing clean and efficient code is a fundamental skill. Clean code is not only easier to understand and maintain, but it also helps to prevent bugs and improves overall program performance. Whether you're a seasoned developer or just starting out, mastering these best practices will elevate your coding game.
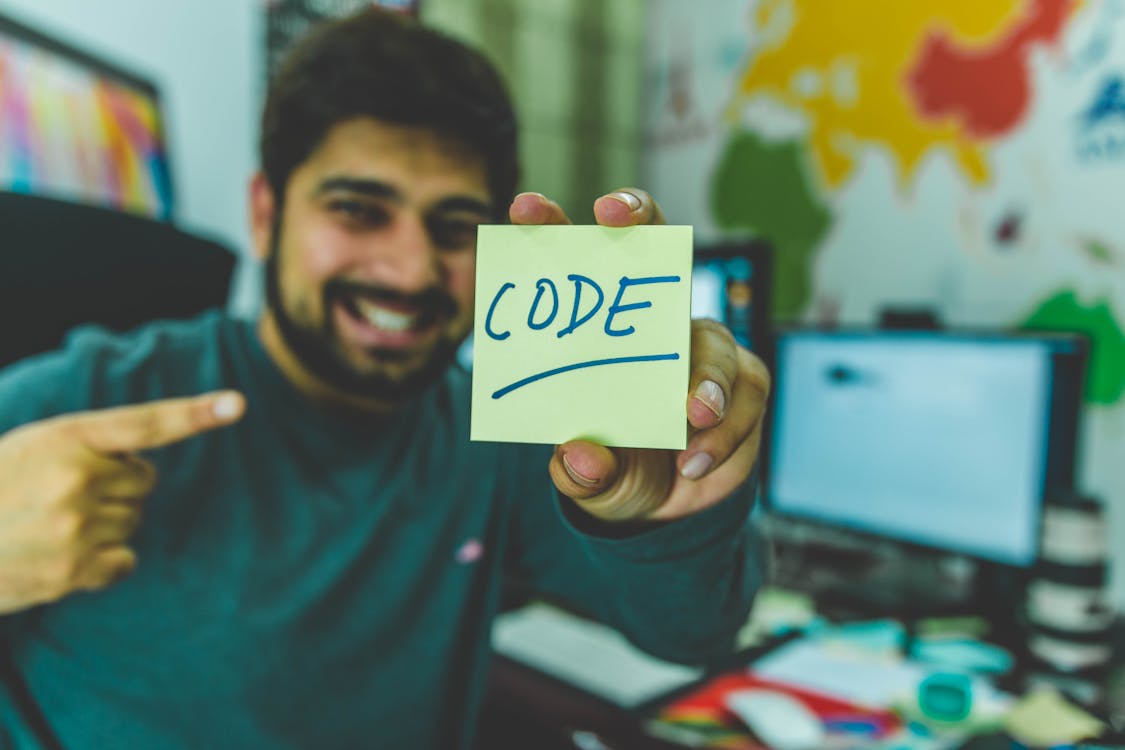
Readability is King:
- Meaningful Names: Descriptive variable and function names are the cornerstone of readable code. Instead of cryptic abbreviations, use names that clearly convey their purpose. (e.g.,
calculate_total_price
vs.calc_totalPrice
). - Clear Formatting: Proper indentation, spacing, and line breaks make your code visually appealing and easier to follow. Consistent formatting improves readability and maintainability.
- Comments, But Not Too Many: Well-placed comments can explain complex logic or non-obvious code sections. However, avoid over-commenting; clean code should be self-documenting whenever possible.
Focus on Functionality:
- Single Responsibility Principle (SRP): Functions should have a single, well-defined purpose. This promotes modularity, reusability, and easier testing. Break down complex functions into smaller, more manageable units.
- Don't Repeat Yourself (DRY): Avoid duplicating code logic. If you find yourself writing the same code block multiple times, refactor it into a reusable function or class. This reduces redundancy and simplifies maintenance.
- Error Handling: Gracefully handle errors and exceptions to prevent unexpected program crashes. Implement proper error checking and informative error messages for debugging purposes.
Embrace Efficiency:
- Algorithmic Complexity: Choose algorithms that are efficient for the task at hand. Consider the time and space complexity of your code to optimize performance.
- Data Structures: Select appropriate data structures for your data types. Using the right data structure can significantly improve the efficiency of your code's operations.
- Profiling: Use profiling tools to identify performance bottlenecks in your code. This allows you to focus optimization efforts on the areas that will have the most significant impact.
Continuous Improvement:
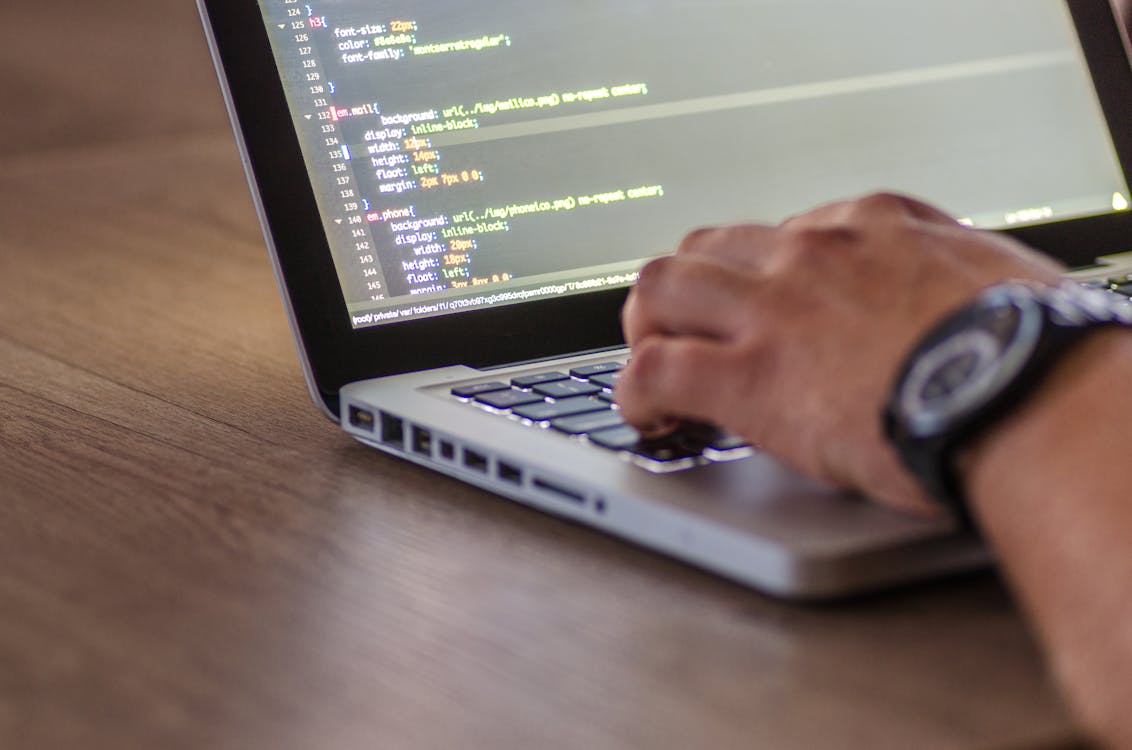
- Testing: Write unit tests to verify the correctness of your code functionality. Unit tests ensure code reliability and facilitate future modifications.
- Version Control: Use a version control system like Git to track changes, revert to previous versions if needed, and collaborate effectively with other developers.
- Refactoring: Regularly review and refactor your code to improve its readability, maintainability, and efficiency. Refactoring helps to keep your codebase clean and adaptable to changing requirements.
You have not logged in, please Login to comment.