Best Practices for Writing Clean Code: A Comprehensive Guide
In the realm of software development, writing clean code is an indispensable skill. Clean code is not just about writing code that works; it's about writing code that is easy to read, maintain, and extend. This article delves into the best practices for writing clean code, aiming to equip developers with the knowledge to improve their coding practices. By adhering to these principles, developers can produce code that is both efficient and understandable, ensuring long-term sustainability and collaboration.
Why Clean Code Matters
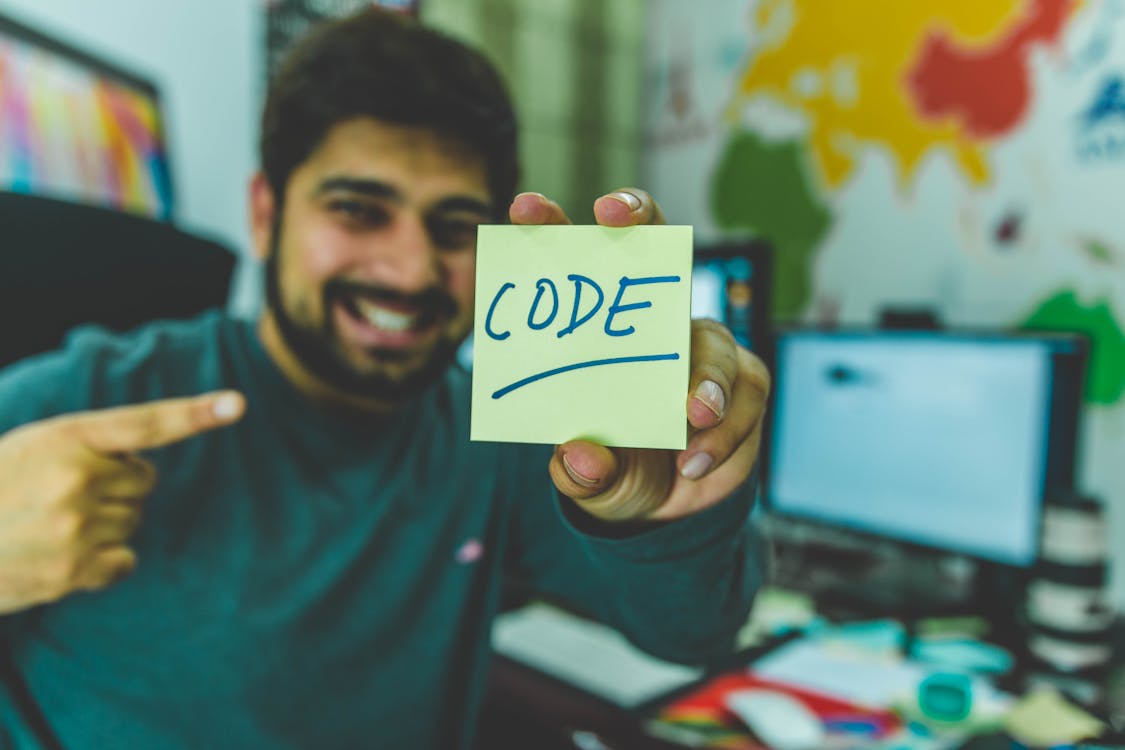
Before diving into specific practices, it's crucial to understand why clean code is so important. Clean code is the backbone of successful software projects for several reasons:
- Readability: Clean code is easy to read and understand. This is crucial for collaboration and for future maintenance by other developers.
- Maintainability: When code is clean, it’s easier to debug and enhance. This leads to faster development cycles and reduced costs.
- Scalability: Clean code can be extended more easily, facilitating the addition of new features without breaking existing functionality.
- Reduced Bugs: Clean, well-structured code tends to have fewer bugs because it's easier to follow and test.
Essential Practices for Writing Clean Code
1. Meaningful Naming Conventions
Using meaningful and descriptive names for variables, functions, and classes is the first step towards writing clean code.
- Variables and Constants: Names should be descriptive enough to convey their purpose. For example, instead of
int a;
, useint numberOfStudents;
. - Functions: Function names should clearly describe what the function does. For example, instead of
void func1();
, usevoid calculateAverageGrade();
. - Classes: Class names should reflect their role in the application. For example,
class DataProcessor;
is better thanclass Processor1;
.
2. Single Responsibility Principle
Every class and function should have a single responsibility. This principle helps in keeping the code modular and easier to understand.
- Functions: Each function should perform a single task. If a function is doing multiple things, consider breaking it into smaller, more focused functions.
- Classes: Similarly, each class should represent a single concept or entity. A class that handles multiple responsibilities should be split into multiple classes.
3. Write Self-Documenting Code
While comments are helpful, the best code is self-explanatory. This means writing code that doesn’t need comments to be understood.
- Clear Logic: Write straightforward and logical code that does not require additional explanations.
- Consistent Naming: Use consistent naming conventions and avoid abbreviations that are not universally understood.
4. Use Comments Wisely
Comments should be used to explain why certain decisions were made, not to explain what the code does. If you find yourself needing to comment on what a piece of code does, it’s a sign that the code could be clearer.
- Explain Why: Use comments to explain the reasoning behind complex or non-obvious code decisions.
- Avoid Redundant Comments: Do not add comments that merely restate what the code is doing.
5. DRY Principle (Don’t Repeat Yourself)
Avoid duplication of code. If you find the same code structure in multiple places, consider refactoring it into a reusable function or method.
- Refactoring: Regularly refactor code to eliminate duplication. This makes the codebase easier to maintain and update.
- Modularization: Break down the code into modules or functions that can be reused throughout the application.
6. Consistent Code Formatting
Consistent formatting makes code easier to read and understand. Use a consistent style for indentation, spacing, and braces.
- Indentation: Use a consistent indentation style throughout the codebase. Typically, four spaces per indentation level is standard.
- Braces: Follow a consistent style for opening and closing braces. For example, always opening braces on the same line or on a new line consistently.
7. Handle Errors Gracefully
Proper error handling is crucial for robust applications. Ensure that your code gracefully handles errors and provides meaningful messages.
- Try-Catch Blocks: Use try-catch blocks to handle exceptions and prevent the application from crashing.
- Meaningful Messages: Provide meaningful error messages that help in diagnosing issues quickly.
8. Write Unit Tests
Unit tests help ensure that individual units of code work as expected. Writing tests can also guide the development process and lead to cleaner code.
- Test Coverage: Aim for high test coverage to ensure that most of the code is tested.
- Automated Testing: Use automated testing tools to run tests frequently and catch issues early.
9. Refactor Regularly
Regular refactoring keeps the codebase clean and manageable. Make it a habit to revisit and improve existing code.
- Code Reviews: Regular code reviews can help identify areas that need refactoring.
- Incremental Improvements: Make small, incremental improvements to the codebase regularly instead of large, disruptive changes.
10. Keep Functions Small
Smaller functions are easier to understand, test, and maintain. Aim to keep functions short and focused on a single task.
- Single Task: Ensure that each function performs only one task.
- Decompose Functions: If a function grows too large, break it down into smaller, more focused functions.
11. Avoid Magic Numbers
Magic numbers are hard-coded values that appear without explanation. Use constants to give these values meaningful names.
- Named Constants: Replace magic numbers with named constants that describe their purpose.
- Centralized Definitions: Define constants in a centralized location to make them easy to manage.
12. Use Design Patterns
Design patterns provide standard solutions to common problems. Familiarize yourself with popular design patterns and use them where appropriate.
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method: Creates objects without specifying the exact class of object that will be created.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified.
Real-World Examples of Clean Code
To better understand these principles, let’s look at some real-world examples of clean code.
Example 1: Meaningful Naming Conventions
Before:
int a = 5;
int b = 10;
int c = a + b;
After:
int numberOfApples = 5;
int numberOfOranges = 10;
int totalFruits = numberOfApples + numberOfOranges;
Example 2: Single Responsibility Principle
Before:
class User:
def __init__(self, name, email):
self.name = name
self.email = email
def send_email(self, message):
# Logic to send email
pass
def save_to_database(self):
# Logic to save user to database
pass
After:
class User:
def __init__(self, name, email):
self.name = name
self.email = email
class EmailService:
def send_email(self, user, message):
# Logic to send email
pass
class UserRepository:
def save_to_database(self, user):
# Logic to save user to database
pass
Tools to Aid in Writing Clean Code
Several tools can help developers maintain clean code:
- Linters: Tools like ESLint for JavaScript, Pylint for Python, and Checkstyle for Java enforce coding standards and identify potential issues.
- Code Formatters: Tools like Prettier automatically format code to a consistent style.
- Static Analysis Tools: Tools like SonarQube analyze code for potential bugs, code smells, and security vulnerabilities.
- Automated Testing Frameworks: Tools like JUnit for Java, PyTest for Python, and Mocha for JavaScript facilitate automated testing.
Conclusion
Writing clean code is a fundamental skill for any developer. It requires a commitment to clarity, simplicity, and continuous improvement. By following the best practices outlined in this guide, developers can produce code that is not only functional but also maintainable, scalable, and easy to understand.
Clean code leads to better collaboration, faster development cycles, and more robust applications. As you continue to hone your coding skills, remember that clean code is a journey, not a destination. Regularly revisit and refine your code to ensure it remains clean and efficient.
You have not logged in, please Login to comment.